Simple jQuery Image SlideShow
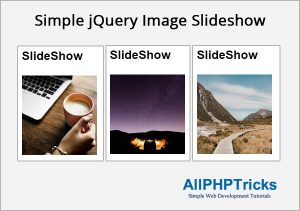
Today I will share how to create simple jQuery image slideshow, there are several plugins available to achieve this goal but i will show you how to do it using jQuery only.
You might like this Simple and Responsive jQuery Carousel Slider.
Steps to Create Simple jQuery Image SlideShow
To create a simple jQuery image slideshow, we need to follow the below steps.
- Write an HTML Markup
- Write CSS
- Include Query Library and Write SlideShow Script
1. Write an HTML Markup
Create an index.html file and paste the following HTML markup in its body section.
<div class="container clearfix">
<div class="box clearfix">
<h2>SlideShow</h2>
<div id="slideshow">
<div class="items active">
<img src="images/pic1.jpg" />
</div>
<div class="items">
<img src="images/pic2.jpg" />
</div>
<div class="items">
<img src="images/pic3.jpg" />
</div>
</div>
</div>
</div> <!-- container End -->
2. Write CSS
Create an style.css file and paste the following styles in it, in the head section.
body {
margin:0px;
font-family:Arial, Helvetica, sans-serif;
}
.clearfix:before, .clearfix:after {
content: "";
display: table;
}
.clearfix:after {
clear: both;
}
.container {
width:1150px;
margin:30px auto;
}
.container .box{
width: 150px;
}
#slideshow {
position:relative;
height:160px;
}
#slideshow div {
position:absolute;
top:0;
left:0;
z-index:8;
visibility:hidden;
}
#slideshow div.active{
z-index:10;
visibility:visible;
}
#slideshow div.last-active{
z-index:9;
visibility:hidden;
}
The above CSS is creating style for our jQuery image slideshow.
3. Include Query Library and Write SlideShow Script
Include jQuery library and write the slideshow script.
<script src="js/jquery.min.js"></script>
<script>
function slideSwitch() {
var $active = $('#slideshow div.active');
if ( $active.length == 0 ) $active = $('#slideshow div:last');
var $next = $active.next().length ? $active.next()
: $('#slideshow div:first');
$active.addClass('last-active');
$next.css({opacity: 0.0})
.addClass('active')
.animate({opacity: 1.0}, 1000, function() {
$active.removeClass('active last-active');
});
}
$(function() {
setInterval( "slideSwitch()", 3000 );
});
</script>
All the images and jQuery library files are available in the download file.
Conclusion
Creating a simple jQuery image slideshow is very simple, as you can see in the above script, we can easily create a beautiful slideshow using jQuery, you can also add additional paragraphs, links along with the image. However to keep this tutorial simple as possible, I only used images.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Thank you for your articles.
You welcome Saima 🙂
Thank you for your articles. 🙂
You welcome 🙂
I like your tutorial
Thanks
Good day Javed,
Thanks for the good tutorial,
I am getting the following error when trying to view the entered data from view.php
Notice: Undefined index: name in C:\xampp\htdocs\view.php on line 50
Dear James,
I am afraid but this tutorial does not contain any file name view.php
I’m new for php and mysqli, i create database name in phpmyadmin, but i don’t know how to handle dbuser name, db password , possible please send to my email id, i learn php from your article only. thanks a lot
Hi Vinoth, i am glad that you found my tutorials helpful. I will be happy if you share my tutorials in your developer circle or social media. Thanks
Username/password is incorrect.
Click here to Login
hii sir im a diploma student im getting this error after login
im not redirecting to the home.php
thank ypu for the code it is very useful
Dear Kajal,
Move the session_start(); at the beginning of each page it exist. Issue will resolved.