Laravel 11 Custom User Registration & Login Tutorial
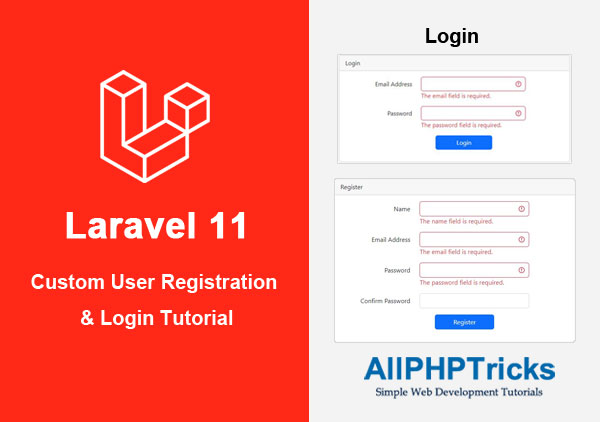
In this tutorial, I will show how to create Laravel 11 custom user registration and login.
By default, Laravel framework comes with a complete user authentication system to cater most applications requirement.
These includes a complete user registration, login and a password reset features. However, sometimes in some projects, you might need a more customized user registration and login system.
In this tutorial, we will learn how to create a custom user registration and login system in Laravel 11.
Readers Also Read: Laravel 11 CRUD Application
Steps to Create Custom User Registration and Login in Laravel 11
- Install & Set Up Laravel 11 App
- Define Custom Registration & Login Routes
- Create a LoginRegister Controller
- Create Login & Registration Blade View Files
Step 1: Install & Set Up Laravel 11 App
First install the Laravel 11 with name CustomLoginRegister using the below command.
composer create-project --prefer-dist laravel/laravel:^11 CustomLoginRegister
Now move to the CustomLoginRegister directory using the below command.
cd CustomLoginRegister
Now create a database with name custom_login_register and configure your database credentials in the .env file like below:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=custom_login_register
DB_USERNAME=your_db_username
DB_PASSWORD=your_db_password
DB_COLLATION=utf8mb4_unicode_ci
By default Laravel 11 comes with the database collation as utf8mb4_0900_ai_ci, which might create an issue when migrating tables to database therefore I also added the DB_COLLATION with value as utf8mb4_unicode_ci in my database credentials to avoid errors.
And then migrate all default tables to database using the below artisan command.
php artisan migrate
Note: Laravel 11 comes with users table, so we don’t need to create a migration or model for it.
Step 2: Define Custom Registration & Login Routes
Now update your routes/web.php file with the following user registration, login, logout and home routes.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\Auth\LoginRegisterController;
Route::controller(LoginRegisterController::class)->group(function() {
Route::get('/register', 'register')->name('register');
Route::post('/store', 'store')->name('store');
Route::get('/login', 'login')->name('login');
Route::post('/authenticate', 'authenticate')->name('authenticate');
Route::get('/home', 'home')->name('home');
Route::post('/logout', 'logout')->name('logout');
});
Step 3: Create a LoginRegister Controller
Create a LoginRegisterController controller using the below artisan command.
php artisan make:controller Auth\LoginRegisterController
A new controller file is created \app\Http\Controllers\Auth\LoginRegisterController.php
It will contain user registration, login and logout functionality.
Now, update your controller file with the following code:
<?php
namespace App\Http\Controllers\Auth;
use App\Http\Controllers\Controller;
use App\Models\User;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use Illuminate\Support\Facades\Hash;
use Illuminate\View\View;
use Illuminate\Http\RedirectResponse;
use Illuminate\Routing\Controllers\HasMiddleware;
use Illuminate\Routing\Controllers\Middleware;
class LoginRegisterController extends Controller implements HasMiddleware
{
public static function middleware(): array
{
return [
new Middleware('guest', except: ['home', 'logout']),
new Middleware('auth', only: ['home', 'logout']),
];
}
public function register(): View
{
return view('auth.register');
}
public function store(Request $request): RedirectResponse
{
$request->validate([
'name' => 'required|string|max:250',
'email' => 'required|string|email:rfc,dns|max:250|unique:users,email',
'password' => 'required|string|min:8|confirmed'
]);
$user = User::create([
'name' => $request->name,
'email' => $request->email,
'password' => Hash::make($request->password)
]);
$credentials = $request->only('email', 'password');
Auth::attempt($credentials);
$request->session()->regenerate();
return redirect()->route('home')
->withSuccess('You have successfully registered & logged in!');
}
public function login(): View
{
return view('auth.login');
}
public function authenticate(Request $request): RedirectResponse
{
$credentials = $request->validate([
'email' => 'required|email',
'password' => 'required'
]);
if(Auth::attempt($credentials))
{
$request->session()->regenerate();
return redirect()->route('home');
}
return back()->withErrors([
'email' => 'Your provided credentials do not match in our records.',
])->onlyInput('email');
}
public function home(): View
{
return view('auth.home');
}
public function logout(Request $request): RedirectResponse
{
Auth::logout();
$request->session()->invalidate();
$request->session()->regenerateToken();
return redirect()->route('login')
->withSuccess('You have logged out successfully!');
}
}
All the above methods in controller are self explanatory, if you still need a detail explanation then check out my Laravel 10 custom user registration and login tutorial.
I have previously explained each method of LoginRegister controller in detail.
Step 4: Create Login & Registration Blade View Files
Now, we need to create our blade view files, these are nothing but simple HTML forms with some bootstrap classes to display a good user registration, login and secured home screens.
Run the below artisan commands to create view files.
php artisan make:view layouts.app
php artisan make:view auth.register
php artisan make:view auth.login
php artisan make:view auth.home
The above commands will create the following files:
- app.blade.php
- register.blade.php
- login.blade.php
- home.blade.php
Update the following code in resources/views/layouts/app.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Laravel 11 Custom User Registration & Login Tutorial - AllPHPTricks.com</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<nav class="navbar navbar-expand-lg bg-light">
<div class="container">
<a class="navbar-brand" href="{{ URL('/') }}">Laravel 11 Login Register</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNavDropdown" aria-controls="navbarNavDropdown" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNavDropdown">
<ul class="navbar-nav ms-auto">
@guest
<li class="nav-item">
<a class="nav-link {{ (request()->is('login')) ? 'active' : '' }}" href="{{ route('login') }}">Login</a>
</li>
<li class="nav-item">
<a class="nav-link {{ (request()->is('register')) ? 'active' : '' }}" href="{{ route('register') }}">Register</a>
</li>
@else
<li class="nav-item dropdown">
<a class="nav-link dropdown-toggle" href="#" role="button" data-bs-toggle="dropdown" aria-expanded="false">
{{ Auth::user()->name }}
</a>
<ul class="dropdown-menu">
<li><a class="dropdown-item" href="{{ route('logout') }}"
onclick="event.preventDefault();
document.getElementById('logout-form').submit();"
>Logout</a>
<form id="logout-form" action="{{ route('logout') }}" method="POST">
@csrf
</form>
</li>
</ul>
</li>
@endguest
</ul>
</div>
</div>
</nav>
<div class="container">
@yield('content')
<div class="row justify-content-center text-center mt-3">
<div class="col-md-12">
<p>Back to Tutorial:
<a href="https://www.allphptricks.com/laravel-11-custom-user-registration-and-login-tutorial/"><strong>Tutorial Link</strong></a>
</p>
<p>
For More Web Development Tutorials Visit: <a href="https://www.allphptricks.com/"><strong>AllPHPTricks.com</strong></a>
</p>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Update the following code in resources/views/auth/register.blade.php
@extends('layouts.app')
@section('content')
<div class="row justify-content-center mt-5">
<div class="col-md-8">
<div class="card">
<div class="card-header">Register</div>
<div class="card-body">
<form action="{{ route('store') }}" method="post">
@csrf
<div class="mb-3 row">
<label for="name" class="col-md-4 col-form-label text-md-end text-start">Name</label>
<div class="col-md-6">
<input type="text" class="form-control @error('name') is-invalid @enderror" id="name" name="name" value="{{ old('name') }}">
@error('name')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="mb-3 row">
<label for="email" class="col-md-4 col-form-label text-md-end text-start">Email Address</label>
<div class="col-md-6">
<input type="email" class="form-control @error('email') is-invalid @enderror" id="email" name="email" value="{{ old('email') }}">
@error('email')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="mb-3 row">
<label for="password" class="col-md-4 col-form-label text-md-end text-start">Password</label>
<div class="col-md-6">
<input type="password" class="form-control @error('password') is-invalid @enderror" id="password" name="password">
@error('password')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="mb-3 row">
<label for="password_confirmation" class="col-md-4 col-form-label text-md-end text-start">Confirm Password</label>
<div class="col-md-6">
<input type="password" class="form-control" id="password_confirmation" name="password_confirmation">
</div>
</div>
<div class="mb-3 row">
<input type="submit" class="col-md-3 offset-md-5 btn btn-primary" value="Register">
</div>
</form>
</div>
</div>
</div>
</div>
@endsection
Update the following code in resources/views/auth/login.blade.php
@extends('layouts.app')
@section('content')
<div class="row justify-content-center mt-5">
<div class="col-md-8">
<div class="card">
<div class="card-header">Login</div>
<div class="card-body">
@if ($message = Session::get('success'))
<div class="alert alert-danger text-center">
{{ $message }}
</div>
@endif
<form action="{{ route('authenticate') }}" method="post">
@csrf
<div class="mb-3 row">
<label for="email" class="col-md-4 col-form-label text-md-end text-start">Email Address</label>
<div class="col-md-6">
<input type="email" class="form-control @error('email') is-invalid @enderror" id="email" name="email" value="{{ old('email') }}">
@error('email')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="mb-3 row">
<label for="password" class="col-md-4 col-form-label text-md-end text-start">Password</label>
<div class="col-md-6">
<input type="password" class="form-control @error('password') is-invalid @enderror" id="password" name="password">
@error('password')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
</div>
<div class="mb-3 row">
<input type="submit" class="col-md-3 offset-md-5 btn btn-primary" value="Login">
</div>
</form>
</div>
</div>
</div>
</div>
@endsection
Update the following code in resources/views/auth/home.blade.php
@extends('layouts.app')
@section('content')
<div class="row justify-content-center mt-5">
<div class="col-md-8">
<div class="card">
<div class="card-header">Home</div>
<div class="card-body">
<div class="alert alert-success">
@if ($message = Session::get('success'))
{{ $message }}
@else
You are logged in!
@endif
</div>
</div>
</div>
</div>
</div>
@endsection
Congratulations! You have developed a custom user registration and login system in Laravel 11. Now you can go ahead and test the app by registering a new user and log in the user in your application.
Run the below artisan command to start Laravel development server.
php artisan serve
Open the following link in web browser.
http://127.0.0.1:8000/register
Conclusion:
In this tutorial, we have learnt how to create a custom user registration and login in Laravel 11. This Laravel custom user login register system enable more customization and flexibility as compared to the Laravel other default authentication systems. If you face any issue in the implementation, feel free to share in the comment section below.
If you found this tutorial helpful, share it with your friends and developers group.
I spent several hours to create this tutorial, if you want to say thanks so like my page on Facebook, Twitter and share it.
Facebook Official Page: All PHP Tricks
Twitter Official Page: All PHP Tricks
Very good post with 100% working code which you can trust.
Javed thank you very much! I will keep visiting your blog.
Dear Panagiotis,
Thanks for your kind comments. Glad to know that you found it useful.